Error #1502: A script has executed for longer than the default timeout period of 15 seconds.
Problem:
You have entered the realm of “Flash Infinite Loop” or at least a really long loop. This is very common when running loops, especially do…while loops. The timeout is now set to 15 seconds (see image below).
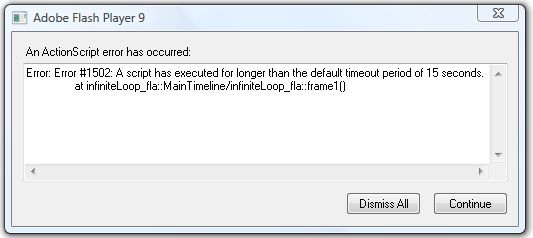
Fix:
Make sure that you are not executing a loop that will never break out. Those poor loops running in circles for all of us Flashers/Flexers.
*******************Warning*******************
The Bad Code below will send your Flex/Flash into and infinite loop and will potentially crash your Development Tool. DO NOT COPY and PASTE the BAD CODE EXAMPLES BELOW.
Bad Code:
var myLength:int = 2;
for (var i:int = 0; i < myLength ; i–);
trace(i);
}
Good Code:
var myLength:int = 2;
for (var i:int = 0; i < myLength ; i++);
trace(i);
}
The code examples above have the var i starting with a number that is smaller than the number it is trying to iterate to. This code basically says as long as i is smaller than myLength then loop and each time I loop make i even smaller.
Bad Code:
*********This code crashed my Flash multiple times.*******
var myArray:Array = [“joe”, “bob”,”pam”];
var randNum:int = Math.round(Math.random()*myArray.length-1);
var curNum:int = 1;
do {
trace(randNum);
} while (randNum == curNum);
The key to using do…while loops in Flash is to make sure that the variable that you are validating against is changed inside the loop itself. Unless the variable that you are validating against changes within the loop itself it will loop forever. This example of a do…while loop is valid but will very likely put Flash into an infinite loop because the likelihood that the number will be 1 is very high, especially considering that the code is rounding and the number 1 is the middle digit of the three. This loop can execute quickly if Flash happens to choose another number or it can loop infinitely. The reason you might be doing this kind of loop is because you want to pull things out of an array, xml, or some other list and don’t want to get duplicates. A better way to do this is to either use the splice() methods in Flash or Flex or if you don’t want to affect the original array you can slice() out a duplicate without the curNum value and then the value will never be repeated because it no longer exists in the items that you are evaluating against. That way you know for sure the item that you don’t want repeated is gone.
There are many other ways to run into an infinite loop. These are just a couple. Feel free to post any questions you have in the comments section and I will answer them promptly.
Thanks and Happy Flashing.