ActionScript Error #1118: Implicit coercion of a value with static type Object to a possibly unrelated type flash.display:DisplayObject
ActionScript Error #1118 can be caused by working with the Display Object. What happens is that as soon as you put a MovieClip, Sprite, etc… into the DisplayObject Flash/Flex thinks that any parent is of Type DisplayObject. Even though you know perfectly well that the object that contains your Sprite is another Sprite or MovieClip Flex and Flash don’t know it. You may have encountered this Flex/Flash Error when you tried to call this.parent
from within a Sprite or Flash MovieClip after using addChild()
(among others) to display or modify the display of a MovieClip/Sprite on the stage. The compiler doesn’t keep track of the Type that the parent is so when you call parent the compiler is confused. Instead it assigns the Type DisplayObject. Your code should still keep working even if you get this error, but there is a reason why the compiler gives you this error. I will show you how to get around this error and how to prevent yourself from getting this error in the future. This AS3 Error can happen any time that you are using any of the following methods:
addChild();
Adds a child DisplayObject instance to this DisplayObjectContainer instance.
addChildAt();
Adds a child DisplayObject instance to this DisplayObjectContainer instance.
contains()
: Determines whether a display object is a child of a DisplayObjectContainer.
getChildAt()
; Returns the child display object instance that exists at the specified index.
getChildByName()
: Retrieves a display object by name.
getChildIndex()
: Returns the index position of a display object.
setChildIndex()
: Changes the position of a child display object.
swap
Children()
: Swaps the front-to-back order of two display objects.
swap
ChildrenAt()
: Swaps the front-to-back order of two display objects, specified by their index values.
Another reason you may get this ActionScript3 Error is by having an external class that is assigned to an object at runtime. Because the class is not predetermined therefore the compiler chokes.
AS3 Error #1118 Solution. You can resolve AS3 Error #1118 by doing one of three things. The first and most highly suggested is to change the way you code. Think from an outside-in perspective when coding rather than an inside-out mentality. The second is to cast the object as the proper Type which explicitly tells the compiler the type of the parent. The third is to just turn off verbose comments in your settings. I don’t suggest doing this last fix as it could lead to many more headaches and is horrible when working with a team.
AS3 Error 1118: Fix #1 – Object Oriented Scripting. Code with an outside-in perspective. This means that instead of the child telling the parent what to do the parent tells the child what to do. This may become tricky if you are completing an animation and need an event to happen when the inner movieClip reaches a certain frame. It is still possible and I may post a tutorial on how to do this with Flash in the near future. But this post is not the right place for it. You can pick up Essential ActionsCript 3
by Collin Moock for a great reference and starting point.
AS3 Error 1118: Fix #2 – Cast the object as the type that you know it is. If you know that it is a MovieClip then tell Flash that it is a MovieClip. You do this by Casting the object as shown below.
Bad Code:
this.parent.doSomething();
Good Code:
MovieClip(this.parent).doSomething();
or
(this.parent as MovieClip).doSomething();
AS3 Error 1118: Fix #3 – *Warning* This method will solve the problem temporarily and allow you to publish your code BUT it will cause you many headaches later. You will not get any warnings or error messages and this is a bad thing I Promise! If you are going to proceed down this path make sure to recheck these boxes after you are done publishing your one problem movie. With that much said, The way you do this is to turn off Strict (Verbose) Error Messages in your preferences as shown below.
Go to File >> Publish Settings >> Click on the “Flash” tab >> Next to “ActionScript version:” click the “Settings…”
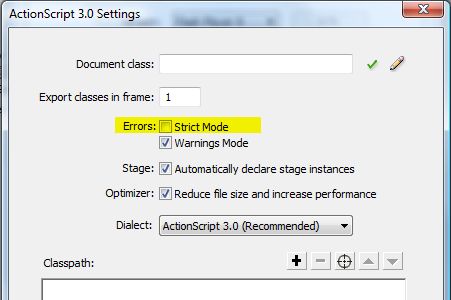
Thanks and as always Happy Flashing,
Curtis J. Morley